In this tutorial you will learn about algorithm and program for quick sort in C.
Quick sort is the fastest internal sorting algorithm with the time complexity O (n log n). The basic algorithm to sort an array a[ ] of n elements can be described recursively as follows:
Quick Sort Algorithm
1. If n < = 1, then return.
2. Pick any element V in a[]. This is called the pivot.
3. Rearrange elements of the array by moving all elements xi > V right of V and all elements xi < = V left of V. If the place of the V after re-arrangement is j, all elements with value less than V, appear in a[0], a[1] . . . . a[j – 1] and all those with value greater than V appear in a[j + 1] . . . . a[n – 1].
4. Apply quick sort recursively to a[0] . . . . a[j – 1] and to a[j + 1] . . . . a[n – 1].
Quick Sort Animation
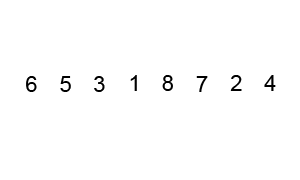
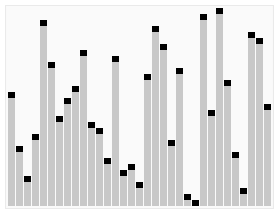
Visualization of the quicksort algorithm. The horizontal lines are pivot values.
Quick Sort Program in C
#include <stdio.h> void quick_sort(int[],int,int); int partition(int[],int,int); int main() { int a[50],n,i; printf("How many elements?"); scanf("%d",&n); printf("\nEnter array elements:"); for(i=0;i<n;i++) scanf("%d",&a[i]); quick_sort(a,0,n-1); printf("\nArray after sorting:"); for(i=0;i<n;i++) printf("%d ",a[i]); return 0; } void quick_sort(int a[],int l,int u) { int j; if(l<u) { j=partition(a,l,u); quick_sort(a,l,j-1); quick_sort(a,j+1,u); } } int partition(int a[],int l,int u) { int v,i,j,temp; v=a[l]; i=l; j=u+1; do { do i++; while(a[i]<v&&i<=u); do j--; while(v<a[j]); if(i<j) { temp=a[i]; a[i]=a[j]; a[j]=temp; } }while(i<j); a[l]=a[j]; a[j]=v; return(j); }
If you found anything incorrect or have any doubts regarding above quick sort in C tutorial then comment below.
can anyone make me understand the implementation of quicksort?
simple programming,easy to understand.
thank you bro.
How to calculate time and space complexity in code?
hi boss can you explain in detail manner how the quick sort is working ?
ofc but first stop calling me boss
call me handsome instead
facts>fiction
If i want to Enter 100 or MORE Number then……
_____
User Want Enter Any Number so..
What he/she will do??
Sir,
you have to return the array in order to print it the array after sorting.And we should sort the key as well i.e,pivot element
So the j in the calling of quicksort equals to j in any of the call
I seriously love the animation part, which makes it more interesting to see the content.
And Sir, you program’s are so, accurate and efficient.
Thank you for providing us this website and helping new coder like me, to get to know, what exactly is a GOOD CODE to approach.
You are seriously doing a good job.
Thank you.
yes,u r right shreya
void quick_sort(int a[],int l,int u)
{
int j;
if(l<u)
{
j=partition(a,l,u);
quick_sort(a,l,j-1);
quick_sort(a,j+1,u);
}
can anyone explain this??
When you take a pivot element and sort all the elements based on that,u need to call quick sort for left group and right group.J is pivot element so here we are calling quicksort for left and right.
Hi admin, i’ve been reading your page for some time and I really like coming back
here. I can see that you probably don’t make money
on your page. I know one interesting method of earning
money, I think you will like it. Search google for:
dracko’s tricks
Sir I just love your content. Keep it up. Wish i could meet you in person and share a hug.
int partition(int a[],int l,int u)
{
int v,i,j,temp;
v=a[l];
i=l;
j=u+1;
do
{
do
i++;
while(a[i]<v&&i<=u);
do
j–;
while(v<a[j]);
if(i<j)
{
temp=a[i];
a[i]=a[j];
a[j]=temp;
}
}while(i<j);
a[l]=a[j];
a[j]=v;
can any one explain me this?
The sorted array is not displaying
We known that their is no keyword to define the array.
I believe partition() has an error. In this loop, it is possible to access a[i] where i > u:
do
{
i++;
}
while(a[i]<v&&i<=u);
On the first call to partition, when u will usually be equal to the last valid index for a[], we will attempt to access memory beyond the array's upper bound. This may crash the program. The solution is simply to reverse the order of the operands in the test:
do
{
i++;
}
while(i<=u&&a[i]<v);
its very useful blog
I think the first do – while block should compare against:
} while( array[i] < v && i < u);
Hey admin, how you got execution time here?
I can’t see any as such
use codeblocks compiler!!
Error found like this ” ) expected ” line 20
awesome cocept
How to reverse the sorted elements in reverse order