String in Java is a sequence of characters. In other programming
languages like C and C++, string is implemented as array of characters while in
Java string is implemented as object of String class.
String class is defined in java.lang package. StringBuffer
and StringBuilder class is also used to create string in Java. We will discuss
about them in upcoming tutorials.
and StringBuilder class is also used to create string in Java. We will discuss
about them in upcoming tutorials.
String objects are immutable i.e. we can’t modify the state
of object. You will learn about String immutability later on in this tutorial.
of object. You will learn about String immutability later on in this tutorial.
How to Create String Object?
String objects can be created in following two ways.
1. By new keyword
2. By string literal
Lets discuss about them one by one.
new Keyword
We can create String object using new keyword in following
way.
way.
String s=new String();
The above code will create an empty String. String class
provides various constructors. An example to create a String initialized by an
array of characters is shown below.
provides various constructors. An example to create a String initialized by an
array of characters is shown below.
char ch[]={'h','e','l','l','o'}; String s=new String(ch);
The above code will create a String object and initialize it
with string “hello”.
with string “hello”.
String Literal
There is an easier way to create string in Java using string
literal. It can be done in following way.
literal. It can be done in following way.
String s1="hello";
Although we are not using new keyword in above example, JVM
will automatically create an object in heap and also create an object in string
constant pool. Now I have introduced a new term string constant pool, lets
discuss about it.
will automatically create an object in heap and also create an object in string
constant pool. Now I have introduced a new term string constant pool, lets
discuss about it.
String Constant Pool
String constant pool is nothing but a special memory area in
heap. Anything written in double quotes (“”) is considered as string literal
and JVM creates an object in string constant pool. Duplicacy is not allowed
in string constant pool. Lets make one java program to understand it.
heap. Anything written in double quotes (“”) is considered as string literal
and JVM creates an object in string constant pool. Duplicacy is not allowed
in string constant pool. Lets make one java program to understand it.
class StringExample { public static void main(String...s) { String s1=new String("hello"); //two objects will be created, one in heap and another in string constant pool String s2="hello"; String s3="hello"; System.out.println("s1==s2 "+(s1==s2)); System.out.println("s2==s3 "+(s2==s3)); } }
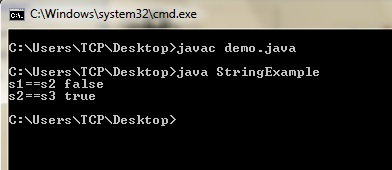
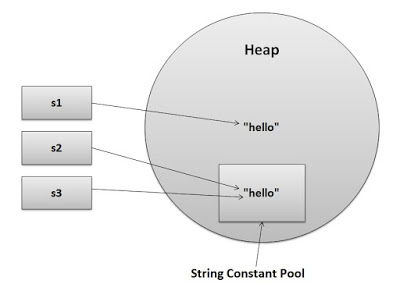
When we create string using string literal, JVM first checks
the string in the string constant pool. If the string is not found in the pool
then JVM creates an object in the pool. Now if we create another string with
the same value then JVM will return the reference of the same object present in
the pool. We can verify this by above program. The reference of s1 and
s2 is not equal because s1 contains the reference of object created in heap
while s2 contains the reference of object created in string constant pool. The
reference of s2 and s3 are equal because they contain the reference of the same
object present in pool.
the string in the string constant pool. If the string is not found in the pool
then JVM creates an object in the pool. Now if we create another string with
the same value then JVM will return the reference of the same object present in
the pool. We can verify this by above program. The reference of s1 and
s2 is not equal because s1 contains the reference of object created in heap
while s2 contains the reference of object created in string constant pool. The
reference of s2 and s3 are equal because they contain the reference of the same
object present in pool.
Why String is Immutable in Java?
As I already told you that String class objects are
immutable. We can’t modify the state of object. Still if we modify it then changes
will not be done in existing object, a new object will be created. Lets take
one example to understand this.
immutable. We can’t modify the state of object. Still if we modify it then changes
will not be done in existing object, a new object will be created. Lets take
one example to understand this.
class StringExample { public static void main(String...s) { String s1="hello"; s1.concat(" world"); //concat method is used to join two strings System.out.println(s1); //will print "hello" } }
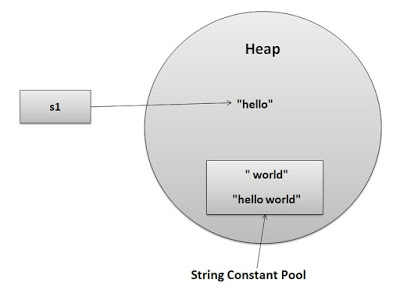
As you can see in the above example that when I have done
changes in the object whose reference is stored in s1, a new object is created
without modifying the existing object. This shows that String is immutable in
java.
Java uses the concept of string literals. Suppose there are
3 reference variables referring to same object. If one reference variable
changes the value of object then it will affect all the reference variables. This
is the reason why String objects are immutable in Java.
3 reference variables referring to same object. If one reference variable
changes the value of object then it will affect all the reference variables. This
is the reason why String objects are immutable in Java.
String immutability
also makes Java secure. String is widely used in storing secure information
like password, so the hackers are not able to do changes in the existing
objects.
In case we want mutable string objects then we can use
StringBuffer and StringBuilder class.
StringBuffer and StringBuilder class.
String Length in Java
Java provides inbuilt method named as length() which allows
us to find the length of a string. An example is given below.
us to find the length of a string. An example is given below.
String s="hello world"; System.out.println(s.length());
The above code will find the length of string “hello world”
i.e. 11.
i.e. 11.
This was all about String in Java. In next tutorials you
will learn about some String class methods. If you find anything incorrect in
above tutorial or if you have any doubts then mention it by commenting below.
will learn about some String class methods. If you find anything incorrect in
above tutorial or if you have any doubts then mention it by commenting below.