Here I will give you code implementation of first come first serve scheduling algorithm in C and C++.
What is FCFS Scheduling Algorithm?
First Come First Served (FCFS) is a Non-Preemptive scheduling algorithm. FIFO (First In First Out) strategy assigns priority to the process in the order in which they request the processor. The process that requests the CPU first is allocated the CPU first. This is easily implemented with a FIFO queue for managing the tasks. As the process comes in, they are put at the end of the queue. As the CPU finishes each task, it removes it from the start of the queue and heads on to the next task.
Let’s take one example to understand this concept.
Assume we have 3 processes, A, B, and C, that arrive in the order given below:
Process | Arrival Time |
A | 0 |
B | 2 |
C | 4 |
And execution time for each process is as given below:
Process | Execution Time |
A | 3 |
B | 2 |
C | 4 |
Now according to the FCFC algorithm, the execution sequence will be as given below:
Process | Arrival Time | Execution Time | Start Time | Finish Time |
A | 0 | 3 | 0 | 3 |
B | 2 | 2 | 3 | 5 |
C | 4 | 4 | 5 | 9 |
Also Read: C Program for Shortest Job First (SJF) Scheduling Algorithm
C Program for FCFS Scheduling
#include<stdio.h>
int main()
{
int n,bt[20],wt[20],tat[20],avwt=0,avtat=0,i,j;
printf("Enter total number of processes(maximum 20):");
scanf("%d",&n);
printf("\nEnter Process Burst Time\n");
for(i=0;i<n;i++)
{
printf("P[%d]:",i+1);
scanf("%d",&bt[i]);
}
wt[0]=0; //waiting time for first process is 0
//calculating waiting time
for(i=1;i<n;i++)
{
wt[i]=0;
for(j=0;j<i;j++)
wt[i]+=bt[j];
}
printf("\nProcess\t\tBurst Time\tWaiting Time\tTurnaround Time");
//calculating turnaround time
for(i=0;i<n;i++)
{
tat[i]=bt[i]+wt[i];
avwt+=wt[i];
avtat+=tat[i];
printf("\nP[%d]\t\t%d\t\t%d\t\t%d",i+1,bt[i],wt[i],tat[i]);
}
avwt/=i;
avtat/=i;
printf("\n\nAverage Waiting Time:%d",avwt);
printf("\nAverage Turnaround Time:%d",avtat);
return 0;
}
C++ Program for FCFS Scheduling
#include<iostream>
using namespace std;
int main()
{
int n,bt[20],wt[20],tat[20],avwt=0,avtat=0,i,j;
cout<<"Enter total number of processes(maximum 20):";
cin>>n;
cout<<"\nEnter Process Burst Time\n";
for(i=0;i<n;i++)
{
cout<<"P["<<i+1<<"]:";
cin>>bt[i];
}
wt[0]=0; //waiting time for first process is 0
//calculating waiting time
for(i=1;i<n;i++)
{
wt[i]=0;
for(j=0;j<i;j++)
wt[i]+=bt[j];
}
cout<<"\nProcess\t\tBurst Time\tWaiting Time\tTurnaround Time";
//calculating turnaround time
for(i=0;i<n;i++)
{
tat[i]=bt[i]+wt[i];
avwt+=wt[i];
avtat+=tat[i];
cout<<"\nP["<<i+1<<"]"<<"\t\t"<<bt[i]<<"\t\t"<<wt[i]<<"\t\t"<<tat[i];
}
avwt/=i;
avtat/=i;
cout<<"\n\nAverage Waiting Time:"<<avwt;
cout<<"\nAverage Turnaround Time:"<<avtat;
return 0;
}
Output:
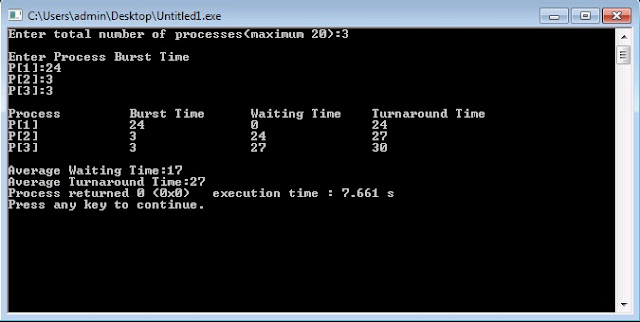%2BScheduling%2BAlgorithm.jpg)
Comment below if you have any queries or suggestions about the above fcfs program in C and C++.
Thanks dude, really helps! 😀
Thanks bro for teaching me
good
Simulate OS as a Resource Allocator and as a Control Program.
nice
It is helpful. But you can do much better. Keep trying. We are with you. JAI HIND !!
thanks very much…..it helped me a lot.Can you please show a program for round robin scheduling algorithm?????
no
Sorry no
google it
Hi, I would like to know that how have you calculated execution time 7.661 seconds at the end. Would you let me know please. Thanks for this post and waiting for your reply.
It is compiler’s inbuilt feature, this execution time also includes the time taken to enter the input.
Thanks for your reply. As you said it’s compiler’s inbuilt feature. I am using visual studio and when I give F5 to run the code, it runs in command prompt. In my case this execution time doesn’t show. I guess you’ll be using different IDE. Can you please give me some information on what you are using to code and run please.
Thanks for your time and valuable reply.
Regards
I am using Dev C++ IDE.
but you’re wrong this program is unable to compute the correct output
How are you implementing FCFS algorithm without considering the arrival times of each process? The waiting time, turn around should be calculated based on the arrival time. I guess you are assuming all of the processes arrive on the same time, at the beginning, but that makes your way of implementing FCFS incorrect. Correct me if I got you wrong 🙂
yep bro ! 🙂 you got that right
the whole program is wrong : the correct implementation would be
#include
#define max 10
// Wap to implement the first come first serve algorithm in operating system.
int main()
{
int n = 0 , i = 0 ;
int pid[max] , a_time[max] , b_time[max] , c_time[max] , w_time[max] , tat_time[max];
float aw_time = 0, atat_time = 0;
printf(“Enter the number of process : “);
scanf(“%d”,&n);
for(i = 0 ; i < n ; i++)
{
pid[i] = i+100 ; // assigned by cpu
}
printf("Enter the arrival time and burst time : ");
for(i = 0 ; i < n ; i++)
{
scanf("%d%d",&a_time[i],&b_time[i]);
}
printf("The input data is \n\n");
printf("Pid\ta_time\tb_time\n");
for(i = 0 ; i < n ; i++)
{
printf("%d\t%d\t%d\n",pid[i],a_time[i],b_time[i]);
}
w_time[0] = 0;
tat_time[0] = b_time[0];
c_time[0] = b_time[0]+a_time[0];
for( i = 1 ; i < n ; i++)
{
c_time[i] = c_time[i-1]+b_time[i];
tat_time[i] = c_time[i] – a_time[i];
w_time[i] = tat_time[i] – b_time[i];
}
for(i = 0 ; i < n ; i++)
{
aw_time = aw_time + w_time[i];
atat_time = atat_time +tat_time[i];
}
printf("\nThe output data is \n");
printf("Pid\ta_time\tb_time\tc_time\ttat_time\tw_time\n");
for(i = 0 ; i < n ; i++)
{
printf("%d\t%d\t%d\t%d\t%d\t\t%d\n",pid[i],a_time[i],b_time[i],c_time[i],tat_time[i],w_time[i]);
}
printf("The average waiting time is %lf and average turn around time is %lf",aw_time/n,atat_time/n);
return 0;
}
But it shows some errors during execution what should we do for that waiting for your rePlay
When we change the order of arrival time ,the output still appears in the ascending order of arrival time
Thanks for your previous two replies. I would like to know that the scheduler which we are using here (FIFO) works for operating system scheduler. While in databases we do have scheduler that runs operators (here processors in FIFO) using FIFO algorithm. I am confused that are database scheduler and operating system scheduler same? If you know something about it will be very helpful to me. I will appreciate for your answer. And many thanks for your educational website.
Sorry I have no idea about it.
Thnx dude
How to explain line by line……any say plz
What if there’s an idle time? How should you do the code? Thanks!
where is arrival time in program
not yet arrived
During its arrival
hi…. how can we calculate the waiting time without knowing the arrival time.. i have not observed the waiting time in the program. any kind of help appreciated.. thanks
i think this program is considering the completion time of previous process is same as the arrival time of the next process… am i correct..?
Thaaaaaaaaaaaaaaaaaaaaaank you .
How do we make a program of FCFS having different arrival time for all other process.
Hint: use if else condtion..
i need this code in c#… do you have..?
Hello Neeraj,
Firstly I would like to thank you for this post.
I have noticed that the average turnaround time “avtat” which gets returned in the output is in decimal format even though you haven’t used any double datatype.
Could you explain ?
Hey I apologise for the mistake I overlooked the system execution time to be average time around time.
Please I need this program in Pascal as soon as possible
I have posted in C#
This code in C# :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication13
{
class Program
{
static void Main(string[] args)
{
int i, j;
int[] burstTime = new int[5];
int[] waitTime = new int[5];
int[] turnaroundTime = new int[5];
int noOfProcess;
float avgWaitTime = 0;
float avgTurnAroundTime = 0;
Console.WriteLine(“Enter Total Process “);
noOfProcess = Convert.ToInt32(Console.ReadLine());
Console.WriteLine(” Enter Process burst time “);
for ( i=0; i<noOfProcess; i++)
{
burstTime[i] = Convert.ToInt32(Console.ReadLine());
}
Console.WriteLine(" Process Burst Time in Sequence ");
for ( i = 0; i < noOfProcess; i++)
{
Console.WriteLine("P["+i+"]"+"="+burstTime[i]);
}
waitTime[0] = 0;
for (i=0;i<noOfProcess;i++)
{
waitTime[0] = 0;
for ( j=0;j<i;j++)
{
waitTime[i] += burstTime[j];
}
}
Console.WriteLine("Process"+"\t\t"+"Burst Time"+"\t\t"+"Waiting Time"+"\t\t"+"Turn Around Time");
for ( i=0;i<noOfProcess;i++)
{
turnaroundTime[i] = burstTime[i] + waitTime[i];
avgWaitTime += waitTime[i];
avgTurnAroundTime += turnaroundTime[i];
}
for (i = 0; i < noOfProcess; i++)
{
Console.WriteLine("P[" + i + "]" + "\t\t" + burstTime[i] + "\t\t" +"\t\t"+ waitTime[i] + "\t\t" + turnaroundTime[i]);
}
avgWaitTime /= i;
avgTurnAroundTime /= i;
Console.WriteLine("Average Waiting Time is :"+avgWaitTime);
Console.WriteLine("Average TurnaroundTime is:"+avgTurnAroundTime);
Console.ReadKey();
}
}
}
where did you code this?
Thaks Bro. Really helped a lot. 🙂
Hi, i would like to ask if there’s an different arrival time? Example if i put 3 processes they user will be ask to input the arrival time, burst time then it will compute the turnaround time and waiting time etc.
yeah there is 😉
thenks bro
thanks sirrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrrr
thanks sirrrrrrrrrrrrr
thank you sir!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
i need help with his homework
Assumptions:
1. All processes are activated at time 0
2. Assume that no process waits on I/O devices.
3. After completing an I/O event, a process is transferred to the ready queue.
4. Waiting time is accumulated while a process waits in the ready queue.
Process Data:
process goes {CPU burst, I/O time, CPU burst, I/O time, CPU burst, I/O time,…….., last CPU burst}
P1 {4,24,5,73,3,31,5,27,4,33,6,43,4,64,5,19,2}
P2 {18,31,19,35,11,42,18,43,19,47,18,43,17,51,19,32,10}
P3 {6,18,4,21,7,19,4,16,5,29,7,21,8,22,6,24,5}
P4 {17,42,19,55,20,54,17,52,15,67,12,72,15,66,14}
P5 {5,81,4,82,5,71,3,61,5,62,4,51,3,77,4,61,3,42,5}
P6 {10,35,12,41,14,33,11,32,15,41,13,29,11}
P7 {21,51,23,53,24,61,22,31,21,43,20}
P8 {11,52,14,42,15,31,17,21,16,43,12,31,13,32,15}
Simulation completed for FCFS (see results in table below).
SJF
FCFS
MLFQ
CPU utilization
82.02%
Avg Wait time (WT)
285.875
Avg Turnaround time (TT)
691.5
Avg Response time (RT)
36.25
SJF CPU utilization:
FCFS CPU utilization: 82.02%
MLFQ CPU utilization:
Thank you, It is better if you take the allocating time from the user..
do you have the code for it??
my code for fcfs:
#include
int main()
{
int p,at[10],bt[90],i,j,wt[100],tat[100],c=0,sum=0;
float avgwt=0;
printf(“enter how many process:”);
scanf(“%d”,&p);
for(i=0;i<p;i++)
{
printf("enter the arrival time of process %d:",i);
scanf("%d",&at[i]);
}
for(i=0;i<p;i++)
{
printf("enter the burst time of process %d:",i);
scanf("%d",&bt[i]);
}
wt[0]=0;
for(i=1;i<p;i++)
{
c=c+bt[i-1];
wt[i]=c-at[i];
}
printf("\n");
for(i=0;i<p;i++)
{
tat[i]=wt[i]+bt[i];
sum=0+wt[i];
}
avgwt=(float)(sum)/p;
printf("arrival\tburst\twait\tturn\n");
for(i=0;i<p;i++){
printf("%d\t%d\t%d\t\%d\n",at[i],bt[i],wt[i],tat[i]);
}
printf("the average wait time is:%d\n",avgwt);
return 0;
}
whats the logic for starting time and finish time (in c)
starting time and finish time (in c)
cpu subrit time arrivel time
P1 6 5
P2 5 0
P3 3 4
Please write this programme in C or C++
avwt and avtat must not intialised int caz they have integer value tooo. so it must be initialised using float in cpp
I need help to with an assignment. I need to create multiprocesses and use different scheduling algorithms to get an optimal output. I am a beginner and a student. , Please email me if you are an expert. henryukwu55 (at) gmail
brother include ariaval time also in your program then it will better ok …
nice bro
Hi Neeraj.
Thanks for your helpful example here. Im trying to program for FCFS.
All related examples online I found are for 1 server (machine) N processes (job).
Like in your example, 3 process would be processed in sequence by one server.
However, in my case, I have several servers (let’s say I have 10 machines) and 100 processes (jobs).
I know about the arrival time of each job, i know how long each job would be finished (all machines have the same speed).
Now I need to get a machine-job match based on FCFS.
The difference of my case with yours is that I cannot assignment all jobs to a single machine.
The constraints in my case is that for each job, it has its own several specific machines MAC to be assignment to. (1 job – 1 machine (this machine should be from MAC).
So in my case, firstly I would make a list of jobs based on their arrival time;
Then I would assign the first job with earlier arrival time to one of its suitable machines;
Do the same to the second coming, the third and more.
I’m new in programming and wondering if you have programmed for similar cases. Any suggestions would be greatly appreciated.
Thanks buddy!!
please explain to me the entire code you’ve use.
explain to me the entire code please.
pls how can i input continue i mean i program it (fcfs) so i want to change the digit that i input instead of me ending the program i want to backspace it and change the digit then program it back and close my program how can i go about it
i don’t know the meaning of awt/ and atat/,but output comes in your program,how it is possible.
then Stop studing. dont waste money and time.
it really helped for our lab session
Heyyy brooo… sooperb, u r oZm.. these articles were very useful for us. Thankyou so much!!! 🙂
stop wasting your time
can u please explain this code execution with code
thank sir this article helped me in cheating during exam
i am hardik gupta topper of IT batch…after got graduation i ll become mahan aadmi
what if arrival time of all processes are diffrent
thanx a lot buddy it help me to bunk the lecture after reading the code as i have to booze around with my imaginary girlfriend ……..
this program is accepting negative numbers as burst time which is physically impossible.try to change that.
Thanks a lot bro!
Grateful from Pakistan.
10q bro,keep up it
how do you compute the process exited?
thanks
PLS I NEED HELP ON A QUESTION.
IMPLEMENT FCFS ALGORITHM USING ANY PROGRAMMING LANGUEGE.
I Need FCFS Algorithm in Pascal Programming Language
Consider a corporate hospital where we have n number of patients waiting for consultation. The amount of time required to serve a patient may vary, say 10 to 30 minutes. If a patient arrives with an emergency, he /she should be attended immediately before other patients, which may increase the waiting time of other patients. If you are given this problem with the following algorithms how would you devise an effective scheduling so that it optimizes the overall performance such as minimizing the waiting time of all patients. [Single queue or multi-level queue can be used]. • Consider the availability of single and multiple doctors • Assign top priority for patients with emergency case, women, children, elders, and youngsters. • Patients coming for review may take less time than others. This can be taken into account while using SJF. a. FCFS b. SJF (primitive and non-pre-emptive ) c. Priority d. Round robin.
plz say how to do this sum
Thankx bro it helped me a lot….
do you have a code in visual basic using visual studio 2017?
Where’s the exe download link? I have no idea on how to create an exe for C++.
my name is optimus prime leader of the autobots
Brother, you helped me a lot and saved me from doing fail in exam.
but I need Shortest Remaining Time First’s C code within 24 hours.
Please make it bro…
Respects from Bangladesh
how to calculate this in fcfs from read file,not key in data.??
Thank you so much it helped me a lot in my lab
ya dude Tq
hmmm Can I ask for Help?
a C++ program instead of turn around time…it should be finish time