Here you will get the shortest job first scheduling program in c with an example.
In the shortest job first scheduling algorithm, the processor selects the waiting process with the smallest execution time to execute next.
Example:
Let’s take one example to understand how SFJ works.
Process | Burst Time |
P1 | 4 |
P2 | 2 |
P3 | 3 |
P4 | 1 |
Now according to the SJF algorithm, the execution sequence of the above processes will be as follow.
Process | Burst Time | Waiting Time | Turnaround Time |
P4 | 1 | 0 | 1 |
P2 | 2 | 1 | 3 |
P3 | 3 | 3 | 6 |
P1 | 4 | 6 | 10 |
Below I have shared the C program for the implementation of the SJF algorithm.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
#include<stdio.h> void main() { int bt[20],p[20],wt[20],tat[20],i,j,n,total=0,pos,temp; float avg_wt,avg_tat; printf("Enter number of process:"); scanf("%d",&n); printf("\nEnter Burst Time:\n"); for(i=0;i<n;i++) { printf("p%d:",i+1); scanf("%d",&bt[i]); p[i]=i+1; //contains process number } //sorting burst time in ascending order using selection sort for(i=0;i<n;i++) { pos=i; for(j=i+1;j<n;j++) { if(bt[j]<bt[pos]) pos=j; } temp=bt[i]; bt[i]=bt[pos]; bt[pos]=temp; temp=p[i]; p[i]=p[pos]; p[pos]=temp; } wt[0]=0; //waiting time for first process will be zero //calculate waiting time for(i=1;i<n;i++) { wt[i]=0; for(j=0;j<i;j++) wt[i]+=bt[j]; total+=wt[i]; } avg_wt=(float)total/n; //average waiting time total=0; printf("\nProcess\t Burst Time \tWaiting Time\tTurnaround Time"); for(i=0;i<n;i++) { tat[i]=bt[i]+wt[i]; //calculate turnaround time total+=tat[i]; printf("\np%d\t\t %d\t\t %d\t\t\t%d",p[i],bt[i],wt[i],tat[i]); } avg_tat=(float)total/n; //average turnaround time printf("\n\nAverage Waiting Time=%f",avg_wt); printf("\nAverage Turnaround Time=%f\n",avg_tat); } |
Output:
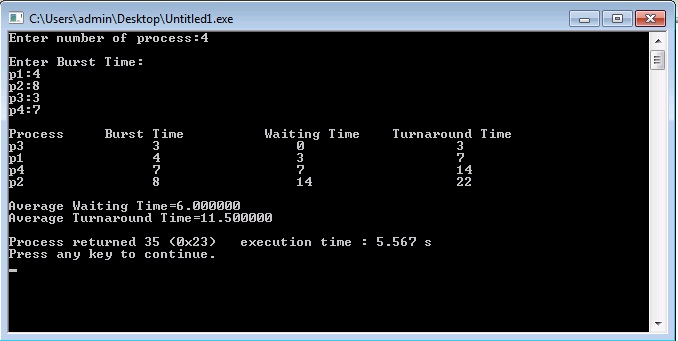%2BScheduling%2BAlgorithm.jpg)
Comment down below for any queries of suggestions related to the SJF algorithm in C.
The last line of the output displays the execution time.
Please provide a code for that.
Revert asap.
Thank you 🙂
no code, just use codeblocks
selection sort algorithm if clause missing
Plz remove code from the text box.
It’s very annoying and difficult to read from that.
plz plz assist me with online saloon management system..thnks
Hi! What help you need with online saloon management system!
Please contact
Just cause it’s simple doesn’t mean it’s not super helulpf.
Convert this program into C++ code please 🙂
i need a program for (preemtive)sjf scheduling algorithm.
pls rply fast
Hello,
I need your help the coding of Identical Parallel Machine in Minimizing Makespan P||Cmax of Longest Processing Time (LPT), Shortest Processing Time (SPT) and First Come First Served (FCFS).
what if the process arrives at different time ex
process arrival time service time
1 0 3
2 2 3
3 3 5
4 4 2
5 8 3
This method does not mention the arrival time of the processes , which is the need of the hour! Please update the concept , otherwise it will be a loss for the whole coding fraternity!
#include
#include
typedef struct
{
int pid;
float at, wt, bt, ta, st;
bool isComplete;
}process;
void procdetail(int i, process p[])
{
printf(“Process id: “);
scanf(“%d”, &p[i].pid);
printf(“Arrival Time: “);
scanf(“%f”, &p[i].at);
printf(“Burst Time: “);
scanf(“%f”, &p[i].bt);
p[i].isComplete = false;
}//procdetail
void sort(process p[], int i, int start)
{
int k = 0, j;
process temp;
for (k = start; k<i; k++)
{
for (j = k+1; j<i; j++)
{
if(p[k].bt < p[j].bt)
continue;
else
{
temp = p[k];
p[k] = p[j];
p[j] = temp;
}
}
}
}//sort
void main()
{
int n, i, k = 0, j = 0;
float avgwt = 0.0, avgta = 0.0, tst = 0.0;
printf("Enter number of processes: ");
scanf("%d",&n);
process p[n];
for (i = 0; i<n; i++)
{
printf("\nEnter process %d's details: ",i);
procdetail(i,p);
}
for (i = 0; i<n; i++)
{
if (p[i].isComplete == true)
continue;
else
{
k = i;
while (p[i].at<=tst && i<n)
i++;
sort (p,i,k);
i = k;
if(p[i].at<=tst)
p[i].st = tst;
else
p[i].st = p[i].at;
p[i].st = tst;
p[i].isComplete = true;
tst += p[i].bt;
p[i].wt = p[i].st – p[i].at;
p[i].ta = p[i].bt + p[i].wt;
avgwt += p[i].wt;
avgta += p[i].ta;
}
}
avgwt /= n;
avgta /= n;
printf("Process Schedule Table: \n");
printf("\tProcess ID\tArrival Time\tBurst Time\tWait Time\tTurnaround Time\n");
for (i = 0; i<n; i++)
printf("\t%d\t\t%f\t%f\t%f\t%f\n", p[i].pid,p[i].at, p[i].bt, p[i].wt, p[i].ta);
printf("\nAverage wait time: %f", avgwt);
printf("\nAverage turnaround time: %f\n", avgta);
}//main
what about if in arrival time is given in the process
Thank you very much..!
I need c++ code?
who can convert it to c++?
Consider a scheduling approach which is non pre-emptive similar to shortest job next in nature. The priority of each job is dependent on its estimated run time, and also the amount of time it has spent waiting. Jobs gain higher priority the longer they wait, which prevents indefinite postponement. The jobs that have spent a long time waiting compete against those estimated to have short run times. The priority can be computed as :
???????? = 1 + ??????? ???? / ????????? ??? ????
Using the data given below compute the waiting time and turnaround time for each process and average waiting time and average turnaround time.
Process Arrival time Burst time
P1 0 20
P2 5 36
P3 13 19
P4 17 42
did you find the answer?if you find it please send to “[email protected]”
if you got the program plz send it to me
useful
and very interesting to read
thank u very much
C++ Program for Shortest Job First (SJF) Scheduling Algorithm
#include
#include
using namespace std;
int main()
{
int bt[20],p[20],wt[20],tat[20],i,j,n,total=0,pos,temp;
float avg_wt,avg_tat;
cout<>n;
cout<<("\nEnter Burst Time:\n");
for(i=0;i<n;i++)
{
cout<<"p"<<i+1<>bt[i];
p[i]=i+1; //contains process number
}
//sorting burst time in ascending order using selection sort
for(i=0;i<n;i++)
{
pos=i;
for(j=i+1;j<n;j++)
{
if(bt[j]<bt[pos])
pos=j;
}
temp=bt[i];
bt[i]=bt[pos];
bt[pos]=temp;
temp=p[i];
p[i]=p[pos];
p[pos]=temp;
}
wt[0]=0; //waiting time for first process will be zero
//calculate waiting time
for(i=1;i<n;i++)
{
wt[i]=0;
for(j=0;j<i;j++)
wt[i]+=bt[j];
total+=wt[i];
}
avg_wt=(float)total/n; //average waiting time
total=0;
cout<<"\nProcess\t Burst Time \tWaiting Time\tTurnaround Time";
for(i=0;i<n;i++)
{
tat[i]=bt[i]+wt[i]; //calculate turnaround time
total+=tat[i];
cout<<"\nP["<<p[i]<<"]\t\t "<<bt[i]<<"\t\t "<<wt[i]<<"\t\t\t"<<tat[i];
}
avg_tat=(float)total/n; //average turnaround time
cout<<"\n\nAverage Waiting Time= "<<avg_wt <<" milliseconds";
cout<<"\nAverage Turnaround Time= "<<avg_tat <<" milliseconds"<<endl;
system("pause");
return 0;
}
Are you ignoring arrival time? i dont see how you kept track of arrival time?
open your eyes
hello. i need a combination program for FCFS and SJF with arrival time.
very usefull,thanku so much
Thank you very much for the code. It helped me in the college practical
sir, will you explain line by line
this program doesnt contain gantt chart and table. we are not satisfied.
solution needed for different arrival time cases
#include
using namespace std;
int main()
{
int n,total,temp,temp1,total1;
cout<>n;
cout<<"\n";
int p[n],b[n],w[n],t[n];
for(int i=0;i<n;i++)
{
p[i]=i+1;
cout<<" Burst time for process "<<i+1<>b[i];
}
for(int i=0;i<n;i++)
{
for(int j=0;jb[j+1])
{
temp=b[j];
b[j]=b[j+1];
b[j+1]=temp;
temp1=p[j];
p[j]=p[j+1];
p[j+1]=temp1;
}
}
}
/* cout<<"\t Process id \t Burst time \n";
for(int i=0;i<n;i++)
{
cout<<"\t "<<p[i]<<" \t\t "<<b[i]<<"\n";
}
*/
w[0]=0;
for(int i=1;i<n;i++)
{
w[i]=w[i-1]+b[i-1];
total=total+w[i];
t[i-1]=w[i-1]+b[i-1];
total1= total1+t[i-1];
}
t[n-1]=w[n-1]+b[n-1];
float avg=(float)total/n;
float avg1=(float)total1/n;
cout<<"\n";
cout<<"_____________________________________________________________________________\n";
cout<<"|\t Process id \t Burst time \t Waiting time \t Turnaround time |\n";
for(int i=0;i<n;i++)
{
cout<<"|\t P"<<p[i]<<" \t\t "<<b[i]<<" \t\t "<<w[i]<<" \t\t "<<t[i]<<"\t\t"<<" |"<<"\n";
}
cout<<"—————————————————————————–\n";
cout<<"\n\t Average waiting time : "<<avg<<"\n";
cout<<" \t Average turnaround time : "<<avg1<<"\n";
}
ohhhhh yaaa
What about the arriving time? What if the user input the arrival time ? How to code that?
not void main()
its int main()
and in last line return 0;
i need a help write a code to fcfs which take in-put directly from the system, i think u got the point
are we calculate the nxt cpu bust time?
for(i=0;i<n;i++)
{
pos=i;
for(j=i+1;j<n;j++)
{
if(bt[j]<bt[pos])
pos=j;
}
temp=bt[i];
bt[i]=bt[pos];
bt[pos]=temp;
temp=p[i];
p[i]=p[pos];
p[pos]=temp;
}
Sir in this loop the temp is used for two time,
sir why we use this for two time
these programs are not executing in my pc , i am using turbo c++.
Could you give me a program sample of SRTF sir?
Why is the i,j ,temp,and pos are used in the program???
Could anyone give its explanation??
Write a C programe using the pipe that perfrom following work:
Parent: Ask two no from user and pass the number to child process.
Child: add the numbers recvied from the parent and pass the result to parent.
Parent: print the table of output received from child.
how to do this program ,,i am not able to do . can anyone help me
can we write this code in circular linked list
Code is not perfectly right in case if mention that arrival time in sense of each process
burst_time[9] = 9999
can anyone explain this line
Sjf executed First lowest value in execution time so using sorting
Sir ye program preemptive hai ya no preemptive
thank you !
Why AT is not given any significance?
This is totally wrong bro
This code is preemptive or non-preemptive??